Google Chrome 115 以降で WebDriver の URL を取得するコードを紹介します.
コード
下記をコピペして貰えば動くと思います.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 |
using System.Net; using System.Text.Json; using Microsoft.Win32; using System.IO.Compression; private static string getChromeVersion() { var node = ""; if (Environment.Is64BitOperatingSystem) { node = @"Wow6432Node\"; } RegistryKey[] keyPathList = new RegistryKey[] { Registry.LocalMachine.OpenSubKey(@"Software\" + node + @"Google\Update\Clients"), Registry.CurrentUser.OpenSubKey(@"Software\" + node + @"Google\Update\Clients"), Registry.LocalMachine.OpenSubKey(@"Software\Google\Update\Clients"), Registry.CurrentUser.OpenSubKey(@"Software\Google\Update\Clients"), }; foreach (var keyPath in keyPathList) { if (keyPath == null) continue; string[] subKeys = keyPath.GetSubKeyNames(); foreach (var subKey in subKeys) { object value = keyPath.OpenSubKey(subKey).GetValue("name"); if (value == null) continue; if (value.ToString().Equals("Google Chrome", StringComparison.InvariantCultureIgnoreCase)) { return keyPath.OpenSubKey(subKey).GetValue("pv").ToString(); } } } return null; } private static string getDriverUrl(string driverInfo, string chromeVersion) { var chromeVersionStripped = String.Join('.',chromeVersion.Split(".").Take(3)) + "."; var driverList = JsonDocument.Parse(driverInfo).RootElement. GetProperty("versions").EnumerateArray().Last(ver => ver.GetProperty("version").ToString().IndexOf(chromeVersionStripped) == 0); var driver = driverList.GetProperty("downloads").GetProperty("chromedriver").EnumerateArray().First(os => os.GetProperty("platform").ToString() == "win32"); return driver.GetProperty("url").ToString(); } private static void updateDriver() { const string DRIVER_INFO_URL = "https://googlechromelabs.github.io/chrome-for-testing/known-good-versions-with-downloads.json"; const string DRIVER_ZIP = "chromedriver.zip"; var chromeVersion = getChromeVersion(); var client = new WebClient(); string driverUrl = getDriverUrl(client.DownloadString(DRIVER_INFO_URL), chromeVersion); client.DownloadFile(driverUrl, DRIVER_ZIP); ZipArchive archive = ZipFile.OpenRead(DRIVER_ZIP); var exeList = archive.Entries.Where(i => i.FullName.Equals("chromedriver-win32/chromedriver.exe")); if (exeList.Count() != 1) { // 何かおかしい return; } exeList.First().ExtractToFile("chromedriver.exe", true); } |
解説
updateDriver()
を呼ぶことで以下の処理を自動的に行います.
- インストールされている Chrome のバージョンの特定.
- 対応する Chrome WebDriver の URL の取得.
- Chrome WebDriver の ZIP アーカイブのダウンロード.
- ZIP アーカイブから Chrome WebDriver の取り出し.
Chrome のバージョンを特定するためにレジストリの読み出しを行うので,NuGet で Microsoft.Win32.Registry
のインストールが必要です.
備考
Selenium バージョン 4.6 以降は,WebDriver の管理を行う Selenium Manager が付属しています.
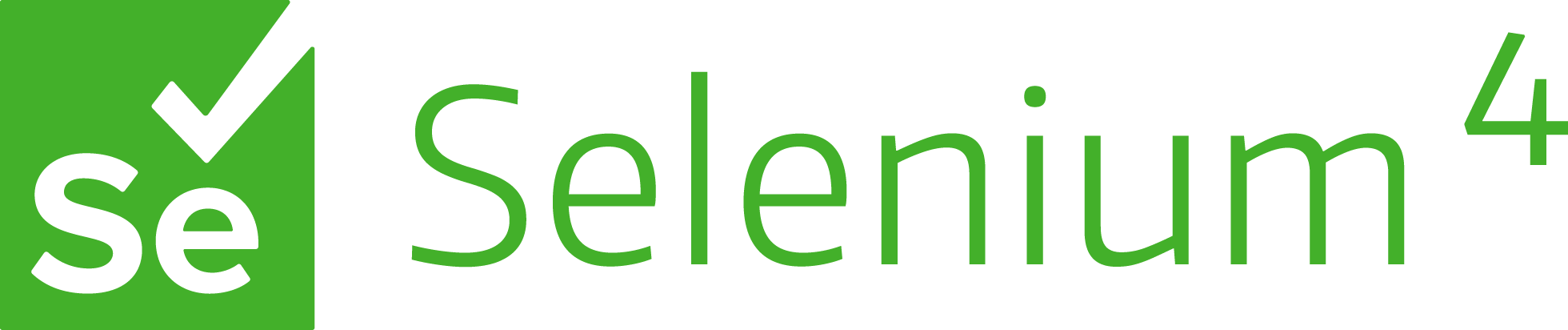
Introducing Selenium Manager
Get a working environment to run Selenium out of the box with a new beta feature!
これを使うと,上のようなコードを書かなくても,下記のようにするだけでドライバーが自動的に更新されます.
1 2 |
using OpenQA.Selenium; using var driver = new OpenQA.Selenium.Chrome.ChromeDriver(); |
ただ,現状の Selenium Manager は CUI コマンドを実行するので,GUI ソフトで使うと一瞬コマンドプロンプトが表示されてしまうという挙動になっています.このあたりの挙動を回避しつつ,WebDriver を自動更新したい場合に,上記のコードが役立つと思います.
コメント